ASCII: 1 byte
Unicode: 2 bytes (or 4 bytes)
Unicode: 2 bytes (or 4 bytes)
Character and Byte Streams
The
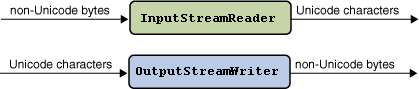
java.io
package provides classes that allow you to convert between Unicode character streams and byte streams of non-Unicode text. With the InputStreamReader
class, you can convert byte streams to character streams. You use the OutputStreamWriter
class to translate character streams into byte streams. The following figure illustrates the conversion process: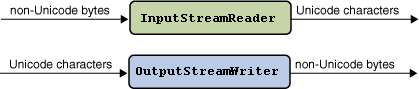